Callback Mechanism
Revel API Gateway supports callbacks. This functionality allow to receive the response to a request in a asynchronous manner.
How does the callback mechanism work?
A typical request/response lifecycle looks like this:
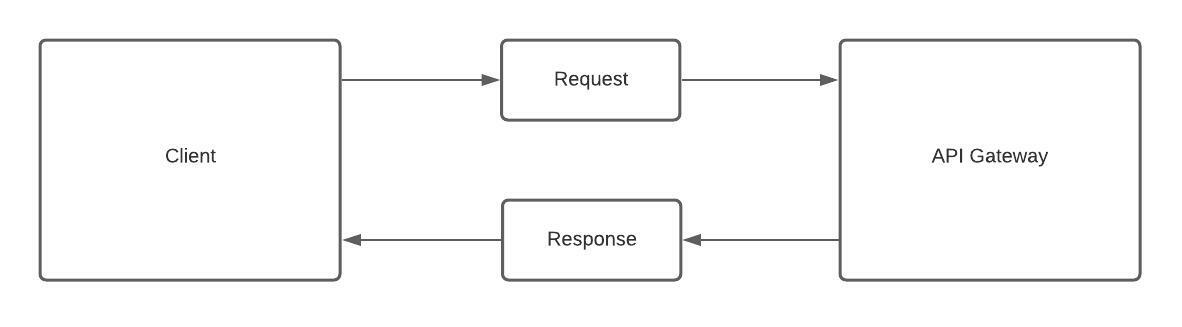
Request/Response model
An HTTP connection is established and a response is sent when it is ready. This is fine for requests that don’t have a long execution time or have a large payload to be delivered. To avoid these drawback, we can use callback mechanism to postpone the response.
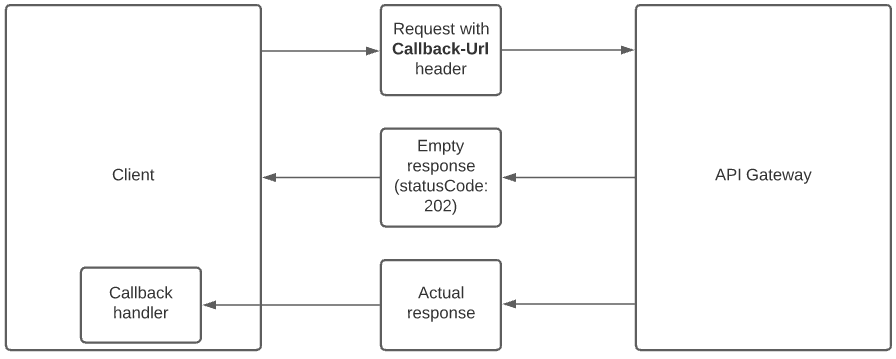
Request/Response model with Callback mechanism
When using callback mechanism, we will get a confirmation response (status code 202 with an empty payload) that indicates, API gateway has accepted our request, but the actual response to the provided request, with the same Correlation-Id
, will be delivered to the callback URL which is provided via HTTP header - Callback-Url
.
When callback mechanism should be used?
Callback mechanism should be used when:
- Response body might be larger than 10MB;
- Request might take longer than 29 seconds (timeout);
- Response should be sent to a different URL than the request;
- Response should be handled in an asynchronous manner;
Preconditions for a client that would like to use callback mechanism
To use callback mechanism, client should implement these functionalities:
- URL that is provided inside the
Callback-Url
should acceptPOST
requests. - URL that is provided inside the
Callback-Url
should return2xx
status code. This will indicate to API Gateway that the response was successfully delivered.
Possible error messages
- When a timeout occurs (request took longer than 29 seconds) API Gateway will return HTTP status code -
504
- When response body exceeds 10MB, API Gateway will return HTTP status code -
413
- When
Callback-Url
is sent for an endpoint that does not support callback mechanism, API Gateway will return HTTP status code -412
Callback mechanism retry logic
If API Gateway fails to deliver the callback response to the client it will:
- Do a maximum of 3 retries;
- Retry if the client returned one of these HTTP status codes:
- 429
- 500
- 502
- 503
- 504
Endpoints that support callback mechanism
Not every endpoint supports a callback mechanism. Here is a list of endpoints that do support it.
"enterprise/Establishment/{anyInt}/menu"
"reports/hourly_sales/json"
"inventory/purchase-orders/create.json"
"reports/customers/json"
"inventory/api/reorder/vendoritems"
"reports/product_mix/data"
"reports/labor/json"
"reports/product_mix/daily/data.xlsx"
"reports/sales_summary/sales_history"
"reports/orders/data.csv"
"resources/MenuProduct"
"reports/orders/json"
"inventoryx/api/inventory-log/Product"
"inventory/purchase-orders/data.json"
"enterprise/Establishment/"
"enterprise/Establishment/{anyInt}/new_list_all"
"reports"
"reports/all_establishment_sales/data.json"
"reports/house_account_summary/json"
"weborders/attributes"
"reports/sales_summary"
"reports/insights_multi_day_hourly_sales"
"reports/void_discount_summary/json"
"weborders/cart_totals"
"reports/operations"
"resources/Modifier/set/{anyInt}"
"reports/labor"
"resources/Customer"
"reports/operations/json"
"reports/voids_summary/json"
"inventoryx/reports/physical_inventory/{anyInt}/finalized-detail-data.json"
"reports/product_mix/daily/data.csv"
"inventory/vendors/items/export"
"inventory/purchase-orders/search-item.json"
"reports/cash_office/json"
"resources/Discount"
"reports/product_mix/pdf"
"reports/payment_summary/json"
"resources/SystemSetting"
"reports/hourly_sales"
"weborders/products"
"resources/Product"
"weborders/menu"
"resources/Permission"
"resources/Modifier"
"reports/product_mix"
"specialresources/cart/submit/"
"resources/ProductModifierClass"
"resources/DeclaredTips"
"resources/VendorOrderableItem"
"resources/BusinessActionLog"
"reports/productcomponent/data.json"
"weborders/v2/products"
"resources/GiftCard"
"resources/OrderItemDataDiff"
"reports/sales_summary/json"
Examples
This section will show examples, how to send a request to API Gateway with and without callback mechanism.
Request without callback mechanism
An example of a request without Callback-Url
using cURL
curl -v --location \
--request GET 'https://api.revelsystems.com/resources/BankDrop' \
--header 'Client-Id: <client-id-goes-here>' \
--header 'Authorization: Bearer <token>'
After we will process your request, we will send a response back with a Correlation-Id
in headers.
< HTTP/1.1 200 OK
< Date: Wed, 06 Oct 2021 11:11:15 GMT
< Connection: keep-alive
< Content-Type: application/json; charset=utf-8
< Content-Length: 120
< Correlation-Id: 9b9c4f01-90e9-4837-90b0-c858dff25f13
<
{
"meta": {
"limit": 20,
"next": null,
"offset": 0,
"previous": null,
"time_zone": null,
"total_count": 0
},
"objects": []
}
Request with callback mechanism
An example of a request with Callback-Url
using cURL
curl -v --location \
--request GET 'https://api.revelsystems.com/resources/BankDrop' \
--header 'Callback-Url: <callback-url-goes-here>' \
--header 'Client-Id: <client-id-goes-here>' \
--header 'Authorization Bearer <token>'
After sending a request to a whitelisted endpoint with Callback-Url
you will receive 202
and a Correlation-Id
in headers.
< HTTP/1.1 202 ACCEPTED
< Date: Wed, 06 Oct 2021 10:53:45 GMT
< Connection: keep-alive
< Content-Type: application/json
< Correlation-Id: a995d20e-8cd5-4ed9-a396-fcd889bf7aaa
< Content-Length: 0
After we will process your request, we will send a POST
request to your provided Callback-Url
where you will see JSON
content.
{
"body": {
"meta": {
"limit": 20,
"next": null,
"offset": 0,
"previous": null,
"time_zone": null,
"total_count": 0
},
"objects": []
},
"method": "GET",
"mimeType": "application/json; charset=utf-8",
"statusCode": 200
}
body
- will contain actual response content without any modificationsmethod
- original method was called by youmimeType
- original type of data we have received during your request processingstatusCode
- original status we have received during your request processing
Correlation-Id
HTTP header inside the response can be mapped with the original request, because the original request response returns the same Correlation-Id
.
Accept-Encoding: gzip, deflate
Accept: */*
Connection: keep-alive
Correlation-Id: a995d20e-8cd5-4ed9-a396-fcd889bf7aaa
Content-Length: 213
Content-Type: application/json
After you will receive a request to your Callback-Url
we expect to receive 2xx
as a response to our request.
Updated about 1 year ago